In this tutorial, we will show you how to implement an autocomplete search box or textbox using jQuery Ui, Ajax with PHP MySQL.
Autocomplete Search Box in PHP MySQL With jQuery UI and Ajax
Here are steps to make the autocomplete search box or textbox in PHP MySQL from the database using jQuery UI and Ajax:
Step 1 – Create a Database Connection
In this step, you will create a file name db.php and update the below code into your file.
The below code is used to create a MySQL database connection in PHP.
<?php $servername='localhost'; $username='root'; $password=''; $dbname = "my_db"; $conn=mysqli_connect($servername,$username,$password,"$dbname"); if(!$conn){ die('Could not Connect MySql Server:' .mysql_error()); } ?>
Step 2 – Create an Autocomplete Search Textbox
In this step, you need to create an autocomplete search box and update the below code into your autocomplete search box in PHP MySQL.
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>Autocomplete Search Box in PHP MySQL - Tutsmake.com</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jqueryui/1.12.1/jquery-ui.css" /> <script src="https://code.jquery.com/jquery-3.4.1.min.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.min.js"></script> <!-- Bootstrap Css --> <link href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" rel="stylesheet"> </head> <body> <div class="container"> <div class="row"> <h2>Search Here</h2> <input type="text" name="term" id="term" placeholder="search here...." class="form-control"> </div> </div> <script type="text/javascript"> $(function() { $( "#term" ).autocomplete({ source: 'ajax-db-search.php', }); }); </script> </body> </html>
After that, don’t forget to update the below-given CSS and JS library in your autocomplete search box form:
<!-- Script --> <script src="https://code.jquery.com/jquery-3.4.1.min.js"></script> <!-- jQuery UI --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jqueryui/1.12.1/jquery-ui.css" /> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.min.js"></script> <!-- Bootstrap Css --> <link href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" rel="stylesheet">
Step 3 – Create a Script to Fetch Autocomplete Data
In this step, you need to create one file name ajax-db-search.php and update the below code into your file.
The below code is to search into a MySQL database table using an Ajax PHP script:
<?php require_once "db.php"; if (isset($_GET['term'])) { $query = "SELECT * FROM users WHERE name LIKE '{$_GET['term']}%' LIMIT 25"; $result = mysqli_query($conn, $query); if (mysqli_num_rows($result) > 0) { while ($user = mysqli_fetch_array($result)) { $res[] = $user['name']; } } else { $res = array(); } //return json res echo json_encode($res); } ?>
Conclusion
In this tutorial, we have learned how to implement an autocomplete search box or textbox in PHP MySQL from the database table using jQuery UI Autocomplete JS.
The php autocomplete textbox from the database example looks like this:
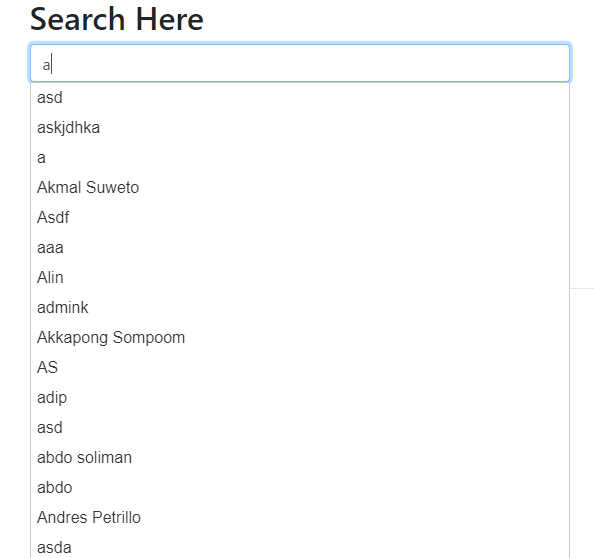
This is a handy, important, and easy example of an Autocomplete Search textbox or input box in PHP MySQL with jQuery UI JS into MySQL.
Recommended PHP Tutorials
If you have any questions or thoughts to share, use the comment form below to reach us.
I copied the code for
“Autocomplete Search Box in PHP MySQL”
and set it up on my shared web server.
It works nicely!
Thanks!
Terry Harris
If i wish to create an autocomplete(product name) textbox with pricing auto retrieval….please advise how should i do?
Works great! How can I make the results clickable?