The Google reCaptcha verification process allows your website or form to be protected from spam and abuse. In this tutorial, we will share with you how to integrate Google reCaptcha validation on php codeigniter form using Google Captcha API.
Codeigniter Google reCaptcha
Here are steps:
- Download Codeigniter Latest
- Set Base URL
- Create Database With Table
- Setup Database Credentials
- Register your website on Google reCaptcha
- Create New Controller
- Create View
- Test This Project
Download Codeigniter Project
In this step, we will download the latest version of Codeigniter, Go to this link Download Codeigniter download the fresh setup of Codeigniter and unzip the setup in your local system.
Set Base URL
Next we will set the some basic configuration on config.php file, so let’s go to application/config/config.php and open this file on text editor.
Set Base URL like this
$config['base_url'] = 'http://localhost/demo/';
Create Database With Table
In this step, we need to create database name demo, so let’s open your phpmyadmin and create the database with the name demo . After successfully create a database, you can use the below sql query for creating a table in your database. We will add some cities with city info.
CREATE TABLE users ( id int(11) NOT NULL AUTO_INCREMENT COMMENT 'Primary Key', name varchar(100) NOT NULL COMMENT 'Name', email varchar(255) NOT NULL COMMENT 'Email Address', contact_no varchar(50) NOT NULL COMMENT 'Contact No', created_at varchar(20) NOT NULL COMMENT 'Created date', PRIMARY KEY (id) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 COMMENT='datatable demo table' AUTO_INCREMENT=1;
Setup Database Credentials
In this step, We need to connect our project to database. we need to go application/config/ and open database.php file in text editor. After open the file in text editor, We need to setup database credential in this file like below.
$db['default'] = array( 'dsn' => '', 'hostname' => 'localhost', 'username' => 'root', 'password' => '', 'database' => 'demo', 'dbdriver' => 'mysqli', 'dbprefix' => '', 'pconnect' => FALSE, 'db_debug' => (ENVIRONMENT !== 'production'), 'cache_on' => FALSE, 'cachedir' => '', 'char_set' => 'utf8', 'dbcollat' => 'utf8_general_ci', 'swap_pre' => '', 'encrypt' => FALSE, 'compress' => FALSE, 'stricton' => FALSE, 'failover' => array(), 'save_queries' => TRUE );
Register your website on Google reCaptcha
Register your website at https://www.google.com/recaptcha/admin/create and get a site key and site secret to add Google recaptcha to your website.
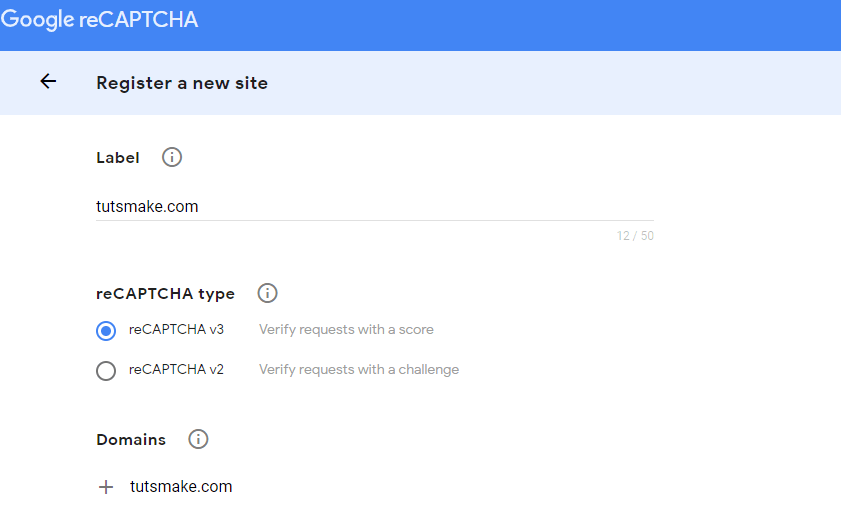
After this, you will get Google ReCaptcha Secret_Site and Secret_Key.

Create New Controller
Create a new Google.php class and methods into it to handle google recaptcha validation process on server side:
<?php defined('BASEPATH') OR exit('No direct script access allowed'); class Google extends CI_Controller { public function __construct() { parent::__construct(); // load model $this->load->helper(array('url','form')); $this->load->library('session'); $this->load->database(); } public function index(){ $this->load->view('google_recaptcha'); } public function googleCaptachStore(){ $data = array('name' => $this->input->post('name'), 'email' => $this->input->post('email'), 'mobile_number' => $this->input->post('mobile_number'), ); $recaptchaResponse = trim($this->input->post('g-recaptcha-response')); $userIp=$this->input->ip_address(); $secret='ENTER_YOUR_SECRET_KEY'; $credential = array( 'secret' => $secret, 'response' => $this->input->post('g-recaptcha-response') ); $verify = curl_init(); curl_setopt($verify, CURLOPT_URL, "https://www.google.com/recaptcha/api/siteverify"); curl_setopt($verify, CURLOPT_POST, true); curl_setopt($verify, CURLOPT_POSTFIELDS, http_build_query($credential)); curl_setopt($verify, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($verify, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($verify); $status= json_decode($response, true); if($status['success']){ $this->db->insert('users',$data); $this->session->set_flashdata('message', 'Google Recaptcha Successful'); }else{ $this->session->set_flashdata('message', 'Sorry Google Recaptcha Unsuccessful!!'); } redirect(base_url('google')); } } ?>
Create View
Create google_catpcha.php view file in application/views/ folder to allow users to check the recaptcha validation on forms:
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <title>Codeigniter Google Recaptcha Form Validation Example - Tutsmake.com</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm" crossorigin="anonymous"> <script src="https://code.jquery.com/jquery-3.2.1.slim.min.js" integrity="sha384-KJ3o2DKtIkvYIK3UENzmM7KCkRr/rE9/Qpg6aAZGJwFDMVNA/GpGFF93hXpG5KkN" crossorigin="anonymous"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.12.9/umd/popper.min.js" integrity="sha384-ApNbgh9B+Y1QKtv3Rn7W3mgPxhU9K/ScQsAP7hUibX39j7fakFPskvXusvfa0b4Q" crossorigin="anonymous"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js" integrity="sha384-JZR6Spejh4U02d8jOt6vLEHfe/JQGiRRSQQxSfFWpi1MquVdAyjUar5+76PVCmYl" crossorigin="anonymous"></script> <script src='https://www.google.com/recaptcha/api.js'></script> <style> .error{ color:red; } </style> </head> <body> <div class="container"> <div class="error"><strong><?=$this->session->flashdata('message')?></strong></div> <br> <br> <div class="row"> <div class="col-md-9"> <form method="post" action="<?php echo base_url('google/googleCaptachStore') ?>"> <div class="form-group"> <label for="formGroupExampleInput">Name</label> <input type="text" name="name" class="form-control" id="formGroupExampleInput" placeholder="Please enter name"> </div> <div class="form-group"> <label for="email">Email Id</label> <input type="text" name="email" class="form-control" id="email" placeholder="Please enter email id"> </div> <div class="form-group"> <label for="mobile_number">Mobile Number</label> <input type="text" name="mobile_number" class="form-control" id="mobile_number" placeholder="Please enter mobile number" maxlength="10"> </div> <div class="g-recaptcha" data-sitekey="YOUR_SITE_KEY"></div> <div class="form-group"> <button type="submit" id="send_form" class="btn btn-success">Submit</button> </div> </form> </div> </div> </div> </body> </html>
Please add your site key in view file:
<div class="g-recaptcha" data-sitekey="YOUR_SITE_KEY"></div>
Test This Project
Go to the browser and hit below the url.
http://localhost/demo/google
Conclusion
In this codeigniter google reCaptcha validation tutorial, We have successfully created a form for showing captcha validation and also we have to call the google api for validating a captcha, after successfully validate a google captcha, we will do next process.
Recommended Tutorials
- Registrtion form validation using jquery validator
- jQuery Form Validation Custom Error Message
- jQuery AJAX Form Submit PHP MySQL
- Simple Registration Form in PHP with Validation
- jQuery Ajax Form Submit with FormData Example
- Google ReCaptcha Form Validation in Laravel
- Codeigniter php jQuery Ajax Form Submit with Validation
If you have any questions or thoughts to share, use the comment form below to reach us.