JavaScript for loop; In this tutorial, you will learn JavaScript for loop with the help of examples.
JavaScript for Loop
- Introduction JavaScript
for
Loop - Syntax of the
for
Loop - Flowchart of
for
loop - JavaScript
for
loop examples- Example 1: Print number from 1 to 5 using for loop
- Example 2: The
for
loop without the initialization part example - Example 3: Sum of 1 to n numbers using for loop in javascript
- Example 4: Find average of n numbers using for loop in JavaScript
Introduction JavaScript for
Loop
The JavaScript for
loop is also known as an entry control loop. The JavaScript for
is test specified condition before executing a block of code. If the condition met true, then block of code will be executed.
Note:- It’s the concise form of loop.
Syntax of the for
Loop
for (initialization; condition; post-expression) { // statements }
Here,
1) initialization
The initialization
expression initializes the loop. The initialization expression is executed only once when the loop starts.
2) condition
The condition
is check once before every iteration. The block of code
inside the loop is executed only when the condition
is met true
. If the condition
metfalse
. The execution of loop will be stopped.
3) post-expression
The for
loop statement also evaluates the post-expression
after each loop iteration. Generally, update post-expression
(increment/decrement).
Flowchart of for
loop
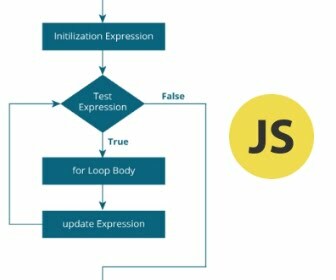
JavaScript for
loop examples
Let’s take some examples of using the for
loop.
Example 1: Print number from 1 to 5 using for loop
The following example uses the javascript for
loop statement that print the numbers from 1 to 5.
<script> for (var count = 1; count <= 5; count++) { document.write(count + "<br>") } </script>
The output of the script shown below:
1 2 3 4 5
How it works:
- First, declare a variable
count
and initialize it to 1. - Next, print the value of
count
. - Increase the value of
count
by 1 in each iteration of the loop.
Example 2: The for
loop without the initialization part example
The following example uses a for
loop that omits the initialization part:
<script> var j = 1; for (; j < 10; j += 2) { document.write(j + "<br>") } </script>
The output of the script shown below:
1 3 5 7 9
Example 3: Sum of 1 to n numbers using for loop in javascript
The following example uses the javascript for
loop statement that sum of 1 to n the numbers.
<script> let sum = 0; let n = 5; for(let i = 1; i <= n; i++) { sum = sum + i; } document.write("Sum of 1 to n number is " + sum+ "<br>") </script>
The output of the script shown below:
Sum of 1 to n number is 15
How it works:
- First, declare a variables
sum and n
and initialize. - Next, specified test condition.
- Increase the value of
count
by 1 in each iteration of the loop. - Using the this formula sum = sum + i;, calculate sum of n number.
- Outside the for loop, Print
sum
variable.
Example 4: Find average of n numbers using for loop in JavaScript
The following example uses the javascript program to find sum of n numbers:
<script> let sum = 0; let n = 5; for(let i = 1; i <= n; i++) { sum = sum + i; } avg = sum/n; document.write("Average of 1 to n number is " + avg + "<br>") </script>
The output of the script shown below:
Average of 1 to n number is 3
How it works:
- First, declare a variables
sum and n
and initialize. - Next, specified test condition.
- Increase the value of
count
by 1 in each iteration of the loop. - Using the this formula sum = sum + i, to calculate sum of n number.
- Outside the loop, use this formula avg = sum/n, to calculate the average of n numbers.
- Outside the for loop, Print
avg
variable.
Conclusion
In this tutorial, you have learned what is for loop and how to use the JavaScript for
loop statements with various examples.