Laravel 8 multiple image upload with validation example; In this tutorial, you will learn how to upload multiple image in laravel 8 app with validation.
Multiple image upload allows the user to select multiple files at once and upload all files to the server. index. html Create a simple HTML page to select multiple files and submit it to upload files on the server. Here, the HTML file contains a form to select and upload files using the POST method.
Before uploading multiple images into database and folder in laravel 8 apps, you can validate image mime type, size, height, width on controller method using this laravel 8 multiple image upload validation tutorial. And as well as, can how to store multiple images in database and folder in laravel 8.
You can see this laravel 8 multiple image upload with validation app in the image below:
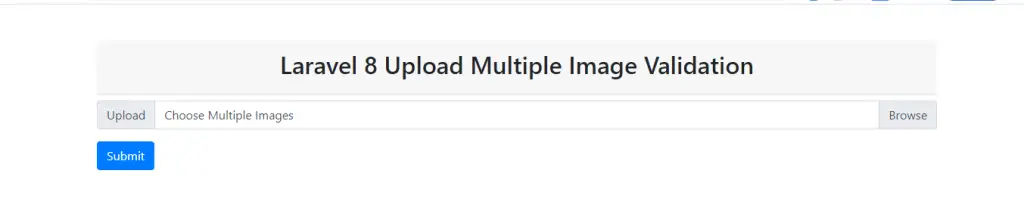
Laravel 8 Multiple Image Upload Validation Example
- Step 1 – Install Laravel 8 Application
- Step 2 – Configure Database In .env File
- Step 3 – Create Photo Model & Migration
- Step 4 – Create Routes
- Step 5 – Create Controller using Artisan Command
- Step 6 – Create Blade View
- Step 7 – Start Development Server
- Step 8 – Start App on Browser
Step 1 – Install Laravel 8 Application
In step 1, open your terminal and navigate to your local web server directory using the following command:
//for windows user cd xampp/htdocs //for ubuntu user cd var/www/html
Then install laravel 8 latest application using the following command:
composer create-project --prefer-dist laravel/laravel blog
Step 2 – Configure Database In .env File
In step 2, open your downloaded laravel 8 app into any text editor. Then find .env file and configure database detail like following:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=db name DB_USERNAME=db user name DB_PASSWORD=db password
Step 3 – Create Photo Model & Migration
In this step, use the below given command to create phtoto migration and model file.
First of all, navigate to project by using the following command:
cd / blog
Then create model and migration file by using the following command:
php artisan make:model Photo -m
The above command will create two files into your laravel 8 multiple image upload with validation tutorial app, which is located inside the following locations:
- blog/app/Models/Photo.php
- blog/database/migrations/create_photos_table.php
So, find create_photos_table.php file inside blog/database/migrations/ directory. Then open this file and add the following code into function up() on this file:
public function up() { Schema::create('photos', function (Blueprint $table) { $table->id(); $table->string('title'); $table->string('path'); $table->timestamps(); }); }
Now, open again your terminal and type the following command on cmd to create tables into your selected database:
php artisan migrate
Step 4 – Create Routes
In this step, open your web.php file, so navigate inside routes directory. Then update the following routes into the web.php file:
use App\Http\Controllers\UploadMultipleImageController; Route::get('multiple-image-upload', [UploadImagesController::class, 'index']); Route::post('multiple-image-upload', [UploadImagesController::class, 'store']);
Step 5 – Create Controller using Artisan Command
In this step, use the below given php artisan command to create controller:
php artisan make:controller UploadImagesController
The above command will create UploadImagesController.php file, which is located inside blog/app/Http/Controllers/ directory.
So open UploadImagesController.php file and add the following code into it:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Photo; class UploadImagesController extends Controller { public function index() { return view('multiple-image-upload'); } public function store(Request $request) { $validateImageData = $request->validate([ 'images' => 'required', 'images.*' => 'mimes:jpg,png,jpeg,gif,svg' ]); if($request->hasfile('images')) { foreach($request->file('images') as $key => $file) { $path = $file->store('public/images'); $name = $file->getClientOriginalName(); $insert[$key]['title'] = $name; $insert[$key]['path'] = $path; } } Photo::insert($insert); return redirect('multiple-image-upload')->with('status', 'Multiple Images has been uploaded successfully'); } }
Step 6 – Create Blade Views
In step this, Navigate to resources/views directory. And then create new blade view file that named multiple-image-upload.blade.php inside this directory.
So, open this multiple-image-upload.blade.php file in text editor and update the following code into it:
<!DOCTYPE html> <html> <head> <title>Uploading Multiple Images In Laravel 8 With Validation</title> <meta name="csrf-token" content="{{ csrf_token() }}"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> </head> <body> <div class="container mt-5"> @if(session('status')) <div class="alert alert-success"> {{ session('status') }} </div> @endif <div class="main"> <div class="card-header text-center font-weight-bold mb-2"> <h2>Laravel 8 Upload Multiple Image Validation</h2> </div> <form name="upload-multiple-image" method="POST" action="{{ url('upload-multiple-image') }}" accept-charset="utf-8" enctype="multipart/form-data"> @csrf <div class="row"> <div class="col-md-12"> <div class="form-group"> <div class="input-group"> <div class="input-group-prepend"> <span class="input-group-text" id="inputGroupFileAddon01">Upload</span> </div> <div class="custom-file"> <input type="file" class="custom-file-input" id="images" name="images[]" multiple=""> <label class="custom-file-label" for="inputGroupFile01">Choose Multiple Images</label> </div> </div> </div> @error('images') <div class="alert alert-danger mt-1 mb-1">{{ $message }}</div> @enderror </div> <div class="col-md-12"> <button type="submit" class="btn btn-primary" id="submit">Submit</button> </div> </div> </form> </div> </div> </body> </html>
Step 7 – Start Development Server
In this step, run the following command on cmd to start development server:
php artisan serve
Step 8 – Start App on Browser
In step this, run this app on browser, so open your browser and fire the following url into browser:
http://127.0.0.1:8000/upload-multiple-image