In this tutorial, you will learn how to create file upload REST API in Node js Express js application for handling multipart/form-data for uploading files.
How to Upload File using Multer with Node Express js Rest Api?
Here are steps:
Step 1 – Set Up Node.js Application
Simply start your cmd or terminal window and run the following commands into it to create node js application or project:
mkdir my-app cd my-app npm init -y mkdir uploads
Step 2 – Install Express and Multer Module
Run the following command on cmd to install express and multer modules:
npm install express multer body-parser --save npm install sharp --save
Step 3 – Create Server.js File and Import Modules
Navigate to node express js project root folder and create server.js file, and then import express, multer, and path dependencies into it; like following:
var express = require("express"); var multer = require('multer'); var bodyParser = require('body-parser'); var app = express(); app.use(express.static(__dirname + '/public')); app.use(bodyParser.urlencoded({ extended: false })); app.use(bodyParser.json()); var storage = multer.diskStorage({ destination: function (req, file, callback) { callback(null, './uploads/'); }, filename: function (req, file, callback) { callback(null, file.originalname); } }); var upload = multer({ storage : storage}).single('file'); app.listen(3000,function(){ console.log("Working on port 3000"); });
Step 4 – Create Route for Rest API to Upload File
To create a file upload route using the REST API, simply create it like the following and add it to the server.js file:
app.post('/file-upload', function (req, res) { upload(req,res,function(err) { if(err) { return res.end("Error uploading file."); } res.end("File is uploaded"); }); });
Step 5 – Start Node Express Js App Server
Run the npm start on cmd or terminal window to start the node express js server:
//run the below command
npm start
Step 6 – File Upload Rest API in Node js using Postman
Simply start your Postman application to upload files using the rest APIs in the node express js project; as shown below picture:
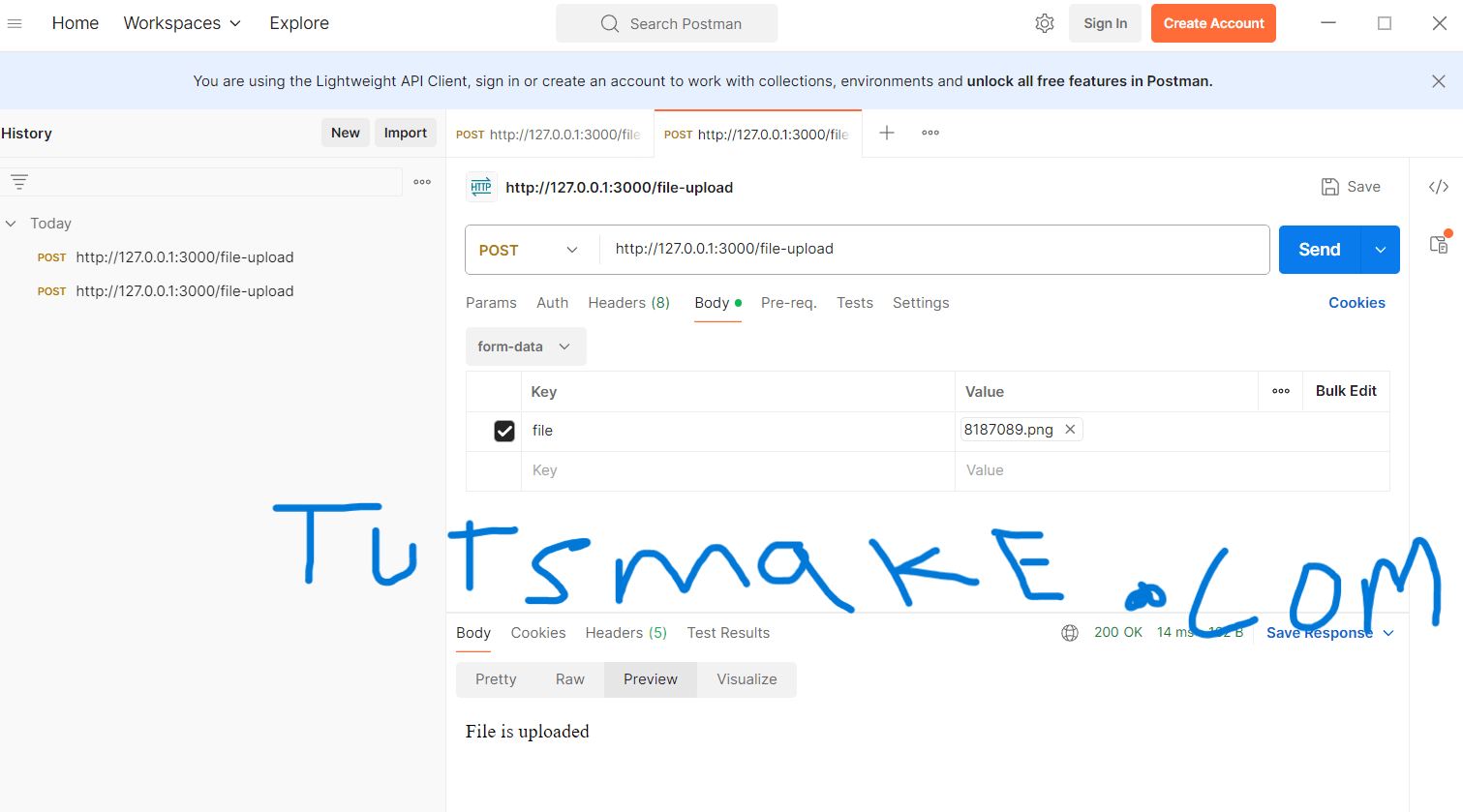
Conclusion
That’s it; You have learned how to create a REST API for uploading files in Node Express JS with Multer.